English
109. Convert Sorted List to Binary Search Tree
Problem Statement
Given the head
of a singly linked list where elements are sorted in ascending order, convert it to a height-balanced binary search tree.
Example 1:
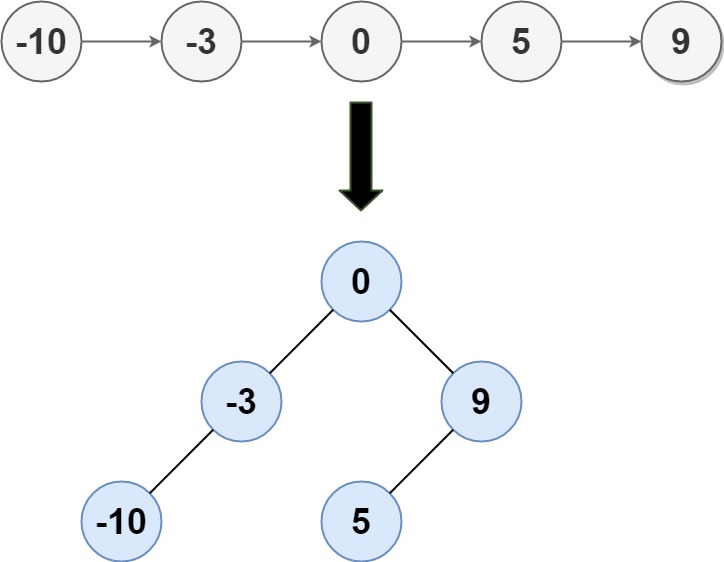
Input: head = [-10,-3,0,5,9]
Output: [0,-3,9,-10,null,5]
Explanation: One possible answer is [0,-3,9,-10,null,5], which represents the shown height balanced BST.
Example 2:
Input: head = []
Output: []
Constraints:
- The number of nodes in
head
is in the range[0, 2 * 104]
. -105 <= Node.val <= 105
Solution:
go
package main
// Definition for singly-linked list.
type ListNode struct {
Val int
Next *ListNode
}
// Definition for a binary tree node.
type TreeNode struct {
Val int
Left *TreeNode
Right *TreeNode
}
func sortedListToBST(head *ListNode) *TreeNode {
if head == nil {
return nil
}
if head.Next == nil {
return &TreeNode{Val: head.Val}
}
slow, fast := head, head
prev := slow
for fast != nil && fast.Next != nil {
prev = slow
slow = slow.Next
fast = fast.Next.Next
}
// slow points to the root of the current subtree
// break the link between the left subtree and the root
prev.Next = nil
root := &TreeNode{Val: slow.Val}
root.Left = sortedListToBST(head)
root.Right = sortedListToBST(slow.Next)
return root
}
...